Recurrences Explained
In this article, you’ll find detailed recurrence information, including data structure and example configuration.
Recurrence datastructure
- DayNumber (int) - day of the month
- DuringWeekday (int) - bitmask for week days
- Sunday = 1,
- Monday = 2,
- Tuesday = 4,
- Wednesday = 8,
- Thursday = 16,
- Friday = 32,
- Every weekday = 62,
- Saturday = 64,
- Weekend days = 65,
- Every day = 127
- Month (int) - month of the year
- WeekOfMonth (int) - number of week of month
- First = 1,
- Second = 2,
- Third = 3,
- Fourth = 4,
- Last = 5
- OccurenceCount (int) - number of occurrences before end
- End (DateTime nullable) - recurrence end date (UTC)
- RecurrenceID (Guid) - recurrence ID
- RecurrenceType (int)
- Daily = 0,
- Weekly = 1,
- Monthly = 2,
- Yearly = 3,
- Minutely = 4,
- Hourly = 5
- Repeat (int) - repeating periodicity
- Range (int) - recurrence end range
- NoEndDate = 0,
- OccurenceCount = 1,
- EndByDate = 2
Recurrence validation messages
-
“Enter recurrence week days.” - Recurrence type is weekly and week days are not provided(DuringWeekday = 0)
-
“Enter recurrence months repeat.” - Recurrence type is monthly and Repeat is 0. (Repeat every nth month with n = 0)
-
“Recurrence month is incorrect” - Recurrence type is yearly or monthly and Month is less than 1 or larger than 12
-
“Recurrence day number is incorrect” - Recurrence type is yearly or monthly and DayNumber is less than 1 or larger than 31
-
“Recurrence end time is incorrect” - Range is End by and End by is not provided or is in the past
-
“Recurrence occurrence count is incorrect” - Range is end after and occurrence count is not provided
-
“Recurrence Range is incorrect.” - Range should be between 0 and 2
-
“Recurrence DuringWeekDay is incorrect.” - Range should be between 0 and 127
-
“Recurrence DayNumber is incorrect.” - Range should be between 1 and 31
-
“Recurrence Month is incorrect.” - Range should be between 1 and 12
-
“Recurrence WeekOfMonth is incorrect.” - Range should be between 0 and 5
-
“Recurrence Repeat is incorrect.” - Raepeat should be 1 or greater
-
“Invalid day of week for a daily recurrence. Only WeekDays. EveryDay, WeekDays. WeekendDays and WeekDays. WorkDays are valid in this context.”
Scenario 1: Daily recurrence with every 3rd day repetition.
var recurrence =
{
DuringWeekday: 127,
Repeat: 3,
RecurreceType: 0,
DayNumber: 1,
Month: 1,
Range: 0 }
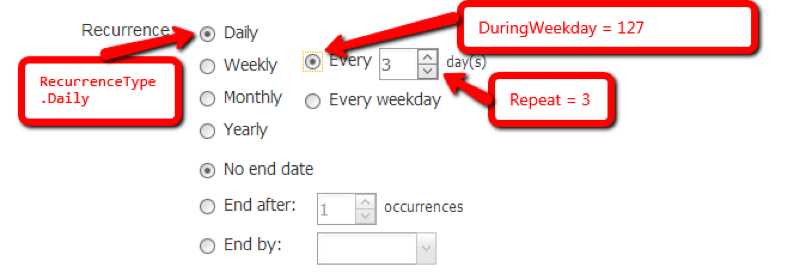
Scenario 2: Daily recurrence on week days (Monday - Friday) with no end date.
var recurrence = {
DuringWeekday: 62,
RecurreceType: 0
DayNumber: 1
Month: 1
Range: 0 }
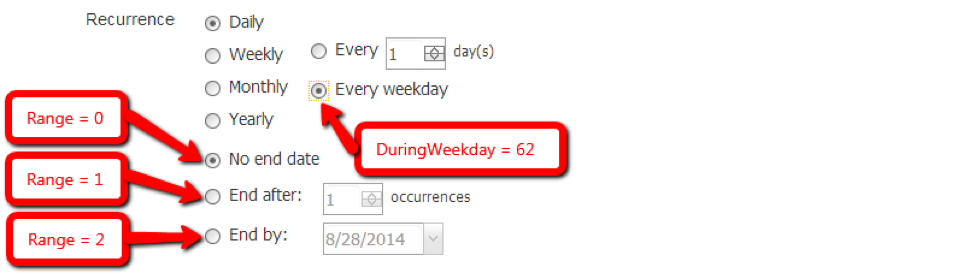
Scenario 3: Monthly recurrence on 12th day on every 3rd month with end date 8/5/2015.
var recurrence = {
DuringWeekday: 1,
RecurreceType: 2,
DayNumber: 12,
Month: 1,
Range: 2,
End: Date(2014, 8, 5)
}
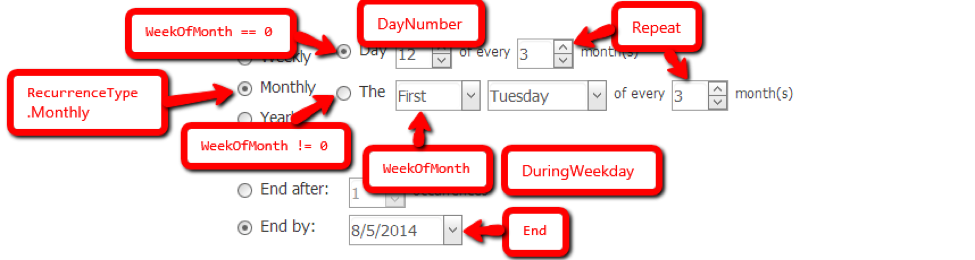
Scenario 4: Weekly recurrence on Tuesday and Wednesday end after 2 occurrences .
var recurrence = {
DuringWeekday: 12,
RecurreceType: 1,
DayNumber: 1,
Month: 1,
Range: 1,
OccurrenceCount: 1
}
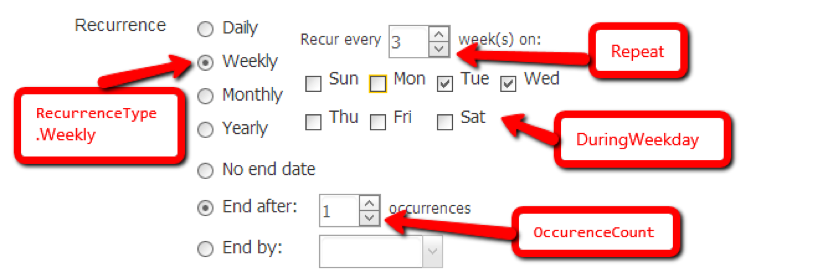
Scenario 5: Yearly recurrence on every January 12th with no end date.
var recurrence = {
DuringWeekday: 1,
RecurreceType: 3,
WeekOfMonth: 0,
DayNumber: 12,
Month: 1,
Range: 0 }
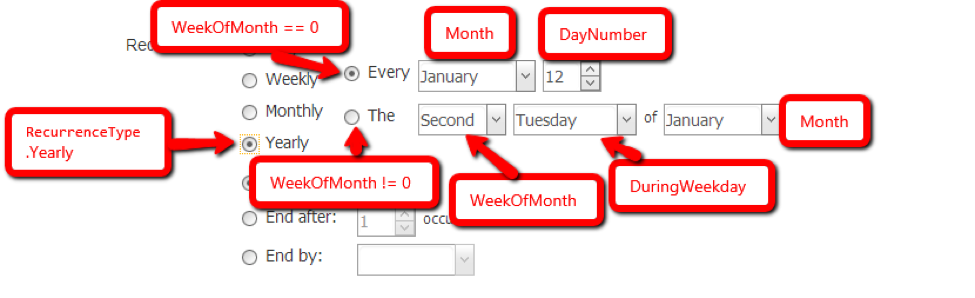